与 word 文档相比,图片格式的文件不需要安装 ms word 就可以直接查看,便于在不同平台之间分享和预览文档内容。而且将 word 文档转换为图片可以保护文档的原始外观,让文档不会因被他人编辑而破坏原有的排布等。本文将展示用 spire.doc for .net 以编程的方式将 word 文档转换为图片的操作方法。
安装 spire.doc for .net
首先,您需要添加 spire.doc for .net 包中包含的 dll 文件作为 .net 项目中的引用。dll 文件可以从此链接下载或通过 安装。
pm> install-package spire.doc
将 word 文档转换为 jpg 格式的图片
spire.doc for .net 为大家提供了 document.savetoimages() 方法以将 word 文档的页面分别转换为位图(bitmap)或图元文件(metafile)。之后,我们可以将位图或图元文件保存为 bmp、emf、jpeg、png、gif、wmf 等格式的图片。以下是将 word 文档转换为 jpg 格式图片的操作步骤。
- 创建 document 类的对象。
- 使用 document.loadfromfile() 方法载入 word 文档。
- 使用 document.savetoimages() 将文档转换为位图集合。
- 在转换出的图片集合中循环,找到指定的图片并保存为 jpg 文件。
- c#
- vb.net
using spire.doc;
using spire.doc.documents;
using system;
using system.drawing;
using system.drawing.imaging;
namespace convertwordtojpg
{
class program
{
static void main(string[] args)
{
//创建 document 类的对象
document doc = new document();
//载入word文档
doc.loadfromfile(@"c:\示例.docx");
//将整个文档的页面分别转换为图片
image[] images = doc.savetoimages(imagetype.bitmap);
//在转换出的图片集合中循环
for (int i = 0; i < images.length; i )
{
//将图片保存的jpg文件
string outputfile = string.format("图片-{0}.jpg", i);
images[i].save(outputfile, imageformat.jpeg);
}
}
}
}
imports spire.doc
imports spire.doc.documents
imports system
imports system.drawing
imports system.drawing.imaging
namespace convertwordtojpg
class program
shared sub main(byval args() as string)
'创建 document 类的对象
dim doc as document = new document()
'载入word文档
doc.loadfromfile("c:\示例.docx")
'将整个文档的页面分别转换为图片
dim images() as image = doc.savetoimages(imagetype.bitmap)
'在转换出的图片集合中循环
dim i as integer
for i = 0 to images.length - 1 step i 1
'将图片保存的jpg文件
dim outputfile as string = string.format("图片-{0}.jpg", i)
images(i).save(outputfile, imageformat.jpeg)
next
end sub
end class
end namespace
将 word 文档转换为 svg 格式的图片
你可以用 spire.doc for .net 将 word 文档保存为一系列字节数组(byte array),然后再将这些字节数组分别写入 svg 文件。以下是将 word 文档转换为 svg 文件的操作步骤。
- 创建 document 类的对象。
- 使用 document.loadfromfile() 方法载入 word 文档。
- 使用 document.savetosvg() 方法将文档保存为字节数组。
- 循环遍历所有字节数组并获取指定的字节数组。
- 将字节数组写入 svg 文件。
- c#
- vb.net
using spire.doc;
using system;
using system.collections.generic;
using system.io;
namespace covnertwordtosvg
{
class program
{
static void main(string[] args)
{
//创建 document 类的对象
document doc = new document();
//载入word文档
doc.loadfromfile(@"c:\示例.docx");
//将文档保存为一系列字节数组
queue svgbytes = doc.savetosvg();
//循环遍历所有字节数组
for (int i = 0; i < svgbytes.count; i )
{
//将数组写入svg文件
byte[][] bytes = svgbytes.toarray();
string outputfile = string.format("图片-{0}.svg", i);
filestream fs = new filestream(outputfile, filemode.create);
fs.write(bytes[i], 0, bytes[i].length);
fs.close();
}
}
}
}
imports spire.doc
imports system
imports system.collections.generic
imports system.io
namespace covnertwordtosvg
class program
shared sub main(byval args() as string)
'创建 document 类的对象
dim doc as document = new document()
'载入word文档
doc.loadfromfile("c:\示例.docx")
'将文档保存为一系列字节数组
dim svgbytes()> as queue
将 word 文档转换为 png 图片并自定义分辨率
分辨率更高的图片能够将内容展示得更清楚,通过以下步骤,你可以在转换 word 文档到 png 图片时,设置其分辨率。
- 创建 document 类的对象。
- 使用 document.loadfromfile() 方法载入 word 文档。
- 使用 document.savetoimages() 将文档转换为位图集合。
- 在位图集合中循环,获取指定的位图。
- 调用自定义的 resetresolution() 方法重新设置图片的分辨率。
- 将位图保存为 png 图片。
- c#
- vb.net
using spire.doc;
using system;
using system.drawing;
using system.drawing.imaging;
using spire.doc.documents;
namespace convertwordtopng
{
class program
{
static void main(string[] args)
{
//创建 document 类的对象
document doc = new document();
//载入 word 文档
doc.loadfromfile(@"c:\示例.docx");
//将整个word文档转换为位图集合
image[] images = doc.savetoimages(imagetype.metafile);
//循环遍历位图集合中的位图
for (int i = 0; i < images.length; i )
{
//设置图片的分辨率
image newimage = resetresolution(images[i] as metafile, 150);
//将图片保存为png文件
string outputfile = string.format("图片-{0}.png", i);
newimage.save(outputfile, imageformat.png);
}
}
//设置图片分辨率
public static image resetresolution(metafile mf, float resolution)
{
int width = (int)(mf.width * resolution / mf.horizontalresolution);
int height = (int)(mf.height * resolution / mf.verticalresolution);
bitmap bmp = new bitmap(width, height);
bmp.setresolution(resolution, resolution);
using (graphics g = graphics.fromimage(bmp))
{
g.drawimage(mf, point.empty);
}
return bmp;
}
}
}
imports spire.doc
imports system
imports system.drawing
imports system.drawing.imaging
imports spire.doc.documents
namespace convertwordtopng
class program
shared sub main(byval args() as string)
'创建 document 类的对象
dim doc as document = new document()
'载入 word 文档
doc.loadfromfile("c:\示例.docx")
'将整个word文档转换为位图集合
dim images() as image = doc.savetoimages(imagetype.metafile)
'循环遍历位图集合中的位图
dim i as integer
for i = 0 to images.length - 1 step i 1
'设置图片的分辨率
dim newimage as image = resetresolution(images(i) as metafile, 150)
'将图片保存为png文件
dim outputfile as string = string.format("图片-{0}.png", i)
newimage.save(outputfile, imageformat.png)
next
end sub
'设置图片分辨率
public shared function resetresolution(byval mf as metafile, byval resolution as single) as image
dim width as integer = ctype((mf.width * resolution / mf.horizontalresolution), integer)
dim height as integer = ctype((mf.height * resolution / mf.verticalresolution), integer)
dim bmp as bitmap = new bitmap(width, height)
bmp.setresolution(resolution, resolution)
imports (graphics g = graphics.fromimage(bmp))
{
g.drawimage(mf, pointeger.empty)
}
return bmp
end function
end class
end namespace
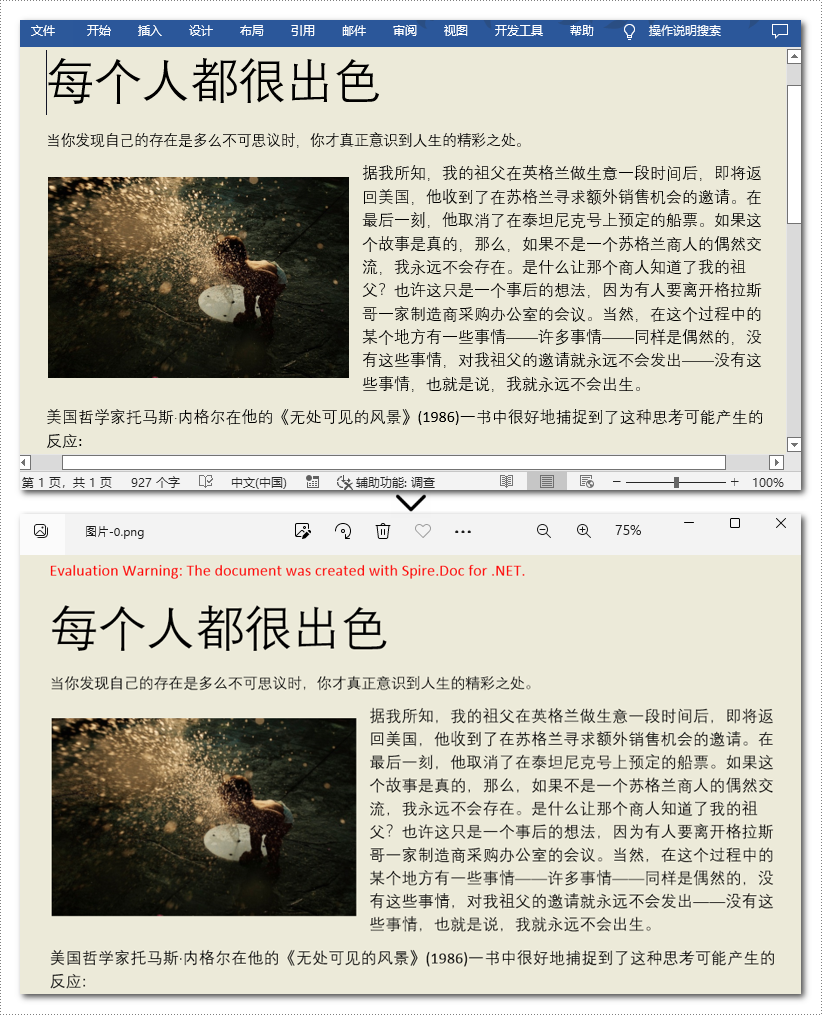
申请临时 license
如果您希望删除结果文档中的评估消息,或者摆脱功能限制,请该email地址已收到反垃圾邮件插件保护。要显示它您需要在浏览器中启用javascript。获取有效期 30 天的临时许可证。